Home / Blog / React 19 Game Changer Hooks
React 19 Game Changer Hooks
3 min read.May 24, 2025
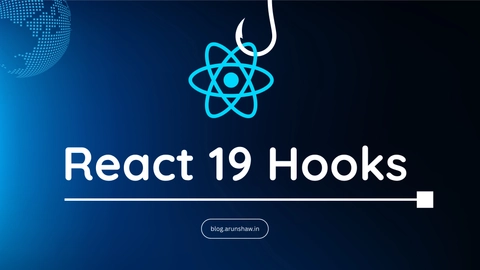
React 19 introduces a powerful set of new and updated Hooks that significantly enhance the developer experience and app performance. These "game changer" Hooks streamline complex logic, reduce lines of code, simplify asynchronous data handling.
use
React introduced the hook named it as use
, The hook can reduce many lines of code in one line only, can handle promises inside it and suspend until the promise resolved and read context from React Context API.
import { use } from 'react';
const getBlogs =() => {
const response = await fetch('https://api.example.com/api/blogs');
const data = await response.json();
return data;
}
const BlogList = () => {
const blogs = use(getBlogs()); //next lines of code will not execute until the api call completed
return blogs.map(blog => <h1 key={comment.id}>{blog.title}</h1>);
}
const Page = () => {
return (
<Suspense fallback={<div> Loading Blogs... </div>}>
<BlogList />
</Suspense>
)
useActionState
Another game changer hook when we need to perform form submissions.
const updateProfile = (data) => {
const response = await fetch(
'https://api.example.com/api/profile',
{
method: 'POST',
body: JSON.stringify(data),
headers: {
'Content-Type':'application/json'
}
}
);
const data = await response.json();
return data;
}
const Profile = ({ name }) => {
const [error, action, isLoading] = useActionState(
async (previousState, formData) => {
await updateProfile(
Object.fromEntries(
formData.entries()
)
)
},
null,
);
return (
<form action={action}>
<input type="text" name="name" defaultValue={name}/>
<button type="submit" disabled={isLoading}> {isLoading ? 'Saving...' : 'Save'} </button>
{error && <p>{error?.message}</p>}
</form>
);
}
useOptimistic
This hook allow you to optimistically update the UI by mutating the data even before the async action completed.
'use client';
import { useOptimistic } from 'react';
const handleCart = (productId, quantity) => {
const response = await fetch(
'https://api.example.com/api/cart',
{
method: 'POST',
body: JSON.stringify(
{
productId: productId,
quantity: quantity
}
),
headers: {
'Content-Type':'application/json'
}
}
);
const data = await response.json();
return data;
}
const CartItem = ({ productId, quantity }) => {
const [optimisticQuantity, setOptimisticQuantity] = useOptimistic(
quantity,
(currentQuantity, nextQuantity) => nextQuantity
);
const handleIncrement = async () => {
setOptimisticQuantity(optimisticQuantity + 1);
// Fires the server action
await updateQuantity(optimisticQuantity + 1);
//if any error occurs it automatically go back to old state
};
return (
<div>
<button onClick={handleIncrement}>
+
</button>
<span>Quantity: {optimisticQuantity}</span>
</div>
);
}
useFormStatus
A simple hook to track form submission status.
import { useFormStatus } from "react-dom";
const SubmitButton = () => {
const status = useFormStatus();
return <button disabled={status.pending}> {status.pending ? 'Saving..' : 'Save'} </button>
}
const App = () => {
const action = (formData) => {
'use server';
const response = await fetch(
'https://api.example.com/api/test',
{
method: 'POST',
body: JSON.stringify(
Object.fromEntries(
formData.entries()
)
),
headers: {
'Content-Type':'application/json'
}
}
);
const data = await response.json();
console.log(data);
}
return (
<form action={action}>
<input type="text" name="name" />
<SubmitButton />
</form>
);
}
Final verdict : With React 19 marks a turning point in how we build for the web, it is scalable, reactive than ever — and this is just the beginning.Learn more about React 19