Home / Blog / Server Side Google OAuth with Nodejs and React
Server Side Google OAuth with Nodejs and React
9 min read.Apr 8, 2025
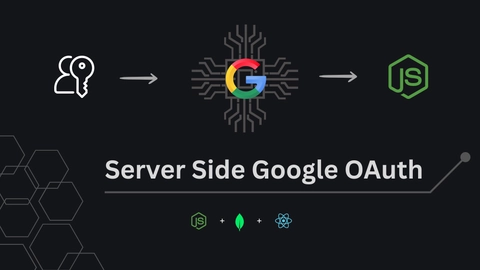
Implement Google sign in functionality in a the React application without any external library, by integrating Google OAuth 2.0 and Google apis directly to our NodeJs application.
Table of Content
Configure Google Consent Screen
Generate Client Id and Client Secret
Initialize a Node Js application
Setup .env file
Building the Application and Integrate Google Apis
Connect with Frontend
Lets Break down what actually happens?
Source Code 😉
Before i start lets see where we should configure the google consent screen
Check here
Configure Google Consent Screen
Visit the above link
Click on select a project and there click on New Project
Give a project name and create a project
Now Click on Create Credentials and select OAuth Client Id
Click on Configure Consent Screen
Select External and Create
Fill the required * fields
Click on Save and Continue
Click on Add or Remove Scopes and Select First two options and click on update
In the next screen click on Add users that you will using for testing purposes
Click an Save and Continue and on the next screen click on back to dashboard.
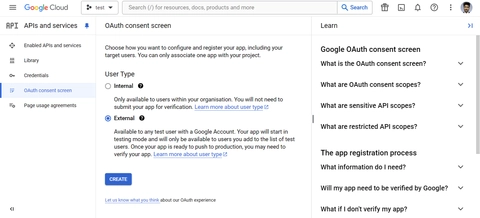
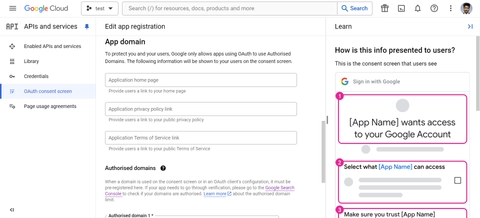
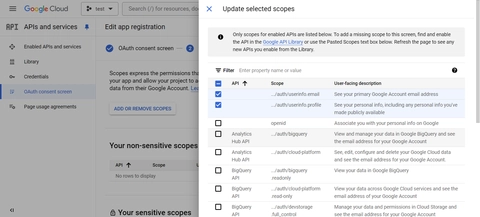
Generate Client Id and Client Secret
Again Click on Create Credentials from top and select OAuth Client Id
Select Web Application
Now follow carefully
Under Authorized redirect URIs we have to add our node js endpoints that google will use to redirect after a successful signin
let put the value
URIs 1 :
http://localhost:8080 / api / oauth / google / success
URIs 2 :
https://YOUR DOMAIN / api/ oauth / google / success
[This is for production use and can be skipped]Click on Create and you can see out client Id and client secret save it somewhere
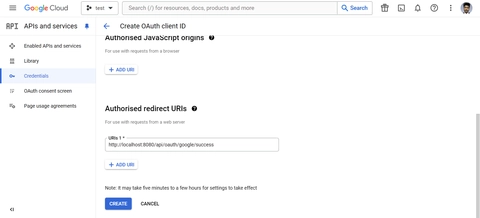
Initialize A Node Js Application
Run npm init -y
Create index.js, .env file, routes folder and controller folder
Run npm i express dotenv jsonwebtoken
Setup .env file
//.env
GOOGLE_CLIENT_ID=YOUR_CLIENT_ID
GOOGLE_CLIENT_SECRET=YOUR_CLIENT_SECRET
REDIRECT_URI=http://localhost:8080/api/oauth/google/success
JWT_SECRET_KEY=YOUR_SECRET_KEY //can be anything
Building the Application and Integrate Google Apis
//package.json
"type": "module"
"scripts" : {
"start": "node index"
}
//Index.js
import express from "express";
import env from 'dotenv';
import { oauthRouter } from "./routes/oauth.route.js";
env.config();
const server = express();
server.use('/api/oauth', oauthRouter);
server.listen(8080, () => {
console.log('Server listening ...')
})
// routes/oauth.route.js
import { Router } from "express";
import { handleGoogleConsentScreen, onSuccessGoogleOAuth, verifyToken, signout } from "../controllers/oauth.controller.js";
const oauthRouter = Router();
oauthRouter.get('/google', handleGoogleConsentScreen);
oauthRouter.get('/google/success', onSuccessGoogleOAuth);
oauthRouter.post('/verify/:token', verifyToken);
oauthRouter.get('/signout', signout);
export { oauthRouter }
/*
* **** Processes involved in implementation of Google OAuth ****
*
* Configure consent screen and get Client Id and Client Secret
* Check: https://console.cloud.google.com/apis/credentials
*
* Creating google consent screen url with client id
* Google redirects the provided redirect uri with a code in params
* Getting access_token and id_token form google apis using the code
* Getting user details using the access token and token id
*/
import jwt from 'jsonwebtoken';
//This funchtion will handle to show google consent screen
const handleGoogleConsentScreen = async (req, res) => {
const url = new URL('https://accounts.google.com/o/oauth2/v2/auth');
const options = {
redirect_uri: process.env.REDIRECT_URI,
client_id: process.env.GOOGLE_CLIENT_ID,
access_type: 'offline',
response_type: 'code',
prompt: 'consent',
scope: [
'https://www.googleapis.com/auth/userinfo.profile',
'https://www.googleapis.com/auth/userinfo.email',
].join(' ')
}
url.search = new URLSearchParams(options);
return res.redirect(url);
}
const getGoogleAccessTokens = async (code) => {
const url = new URL('https://oauth2.googleapis.com/token');
const tokenExchangeOptions = {
code,
client_id: process.env.GOOGLE_CLIENT_ID,
client_secret: process.env.GOOGLE_CLIENT_SECRET,
redirect_uri: process.env.REDIRECT_URI,
grant_type: 'authorization_code',
}
url.search = new URLSearchParams(tokenExchangeOptions);
let response = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
}
})
let data = await response.json();
return data;
}
//This funchtion will be to get userinfo by using acces_token and id_token
const getGoogleUserInfo = async (access_token, id_token) => {
const url = new URL('https://www.googleapis.com/oauth2/v1/userinfo');
const options = {
alt: 'json',
access_token
}
url.search = new URLSearchParams(options);
let response = await fetch(url, {
headers: {
Authorization: `Bearer ${id_token}`,
},
})
let data = await response.json();
return data;
}
const onSuccessGoogleOAuth = async (req, res) => {
const { code } = req.query;
try {
let { access_token, id_token } = await getGoogleAccessTokens(code);
let data = await getGoogleUserInfo(access_token, id_token);
let sessionToken = jwt.sign(data, process.env.JWT_SECRET_KEY);
const date = new Date();
date.setDate(date.getDate() + 30);
res.cookie('session', sessionToken, {
path: '/',
expires: date,
domain: process.env.COOKIE_DOMAIN,
})
return res.redirect(process.env.FRONTEND_URL);
} catch (error) {
return res.redirect(`${process.env.FRONTEND_URL}?error=Something wen wrong`);
}
}
const verifyToken = (req, res) => {
const { token } = req.params;
try {
let validation = jwt.verify(token, process.env.JWT_SECRET_KEY);
return res.send({ validation });
} catch (error) {
return res.status(404).send({ validation: null });
}
}
const signout = (req, res) => {
res.clearCookie('session', { domain: process.env.COOKIE_DOMAIN });
return res.redirect(process.env.FRONTEND_URL);
}
export { handleGoogleConsentScreen, onSuccessGoogleOAuth, verifyToken, signout }
Now our nodejs endpoints are ready. lets connect it to our frontend and i will be using nextjs, a react framework
Connect with Frontend
Initialize a next app with
npx create-next-app
Now lets create a simple button to signin with google
// app/page.js
import Link from 'next/link'
import styles from './page.module.css'
import { cookies } from 'next/headers';
const getSession = async () => {
let token = cookies().get('session')?.value;
let response = await fetch(`http://localhost:8080/api/oauth/verify/${token}`);
let { validation } = await response.json();
return validation;
}
export default async function Home() {
let user = await getSession();
return (
<main className={styles.main}>
{user ? (
<div>
<img src={user.picture} alt={user.name} />
<h2> {user.name} </h2>
<p> {user.email} </p>
<Link href={'http://localhost:8080/api/oauth/signout'}>
<button> Signout </button>
</Link>
</div>
) : (
<Link href={'http://localhost:8080/api/oauth/google'}>
<button> Signin with Google </button>
</Link>
)}
</main>
)
}
Let's Break down what actually happens?
We have configured the google signin success url with our node js end point
So on success google will send a code in query param our end point
Like this Redirect_URL?code=y8qy***
We have to exchance this code by calling google apis to get the user data
After getting the userdata we are creating a token with json web token
Now we are setting a cookie with a token as a value in our frontend application and redirecting to it
From the frontend we are getting that cookie from cookie storage
Then we are hitting /oauth/verify endpoint to to get the userdata by decoding the tokenThrough this, the session will persist till the the time of the cookie expiration
Source Code 😉
Check here
Thank you for joining me with the journey of Google OAuth integration with React and Node js. Hope this blog helps you a lot.